前回つくったタブパネルスクリプトをもとにして、
jQueryプラグインについて
前回の始めに言及したように、
例えば、
jQuery(function(){
$('div.tabArea').tabPanel();
// 対象のjQueryオブジェトに対してメソッドを実行する
});
$('div.
function関数とメソッド
function関数
まずはメソッドについておさらいをしましょう。JavaScriptでは、
function sample(){
alert('Hello world');
}
sample(); // 実行する
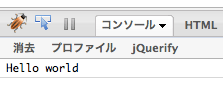
メソッド
メソッドとは、
例をあげてみます。
(function($){
$.fn.sample = function(){
console(this);
}
})(jQuery);
jQuery(function($){
$('div').sample(); // メソッドを実行する
});
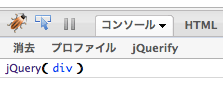
jQuery(function($){ ~ });と(function($){ ~ })(jQuery);
jQuery(function($){ ~ });
jQueryのメソッドや処理を実行する場合は、
(function($){ ~ })(jQuery);
カプセル化
実行するものはjQuery(function($){});にまとめて入れてしまえばいいのですが、
タブパネルスクリプトの関数化とメソッド化
前回作ったスクリプトは以下ですが
jQuery(function($){
var tabArea = $('div.tabArea');
var tabPanel = $('div.tabPanel',tabArea);
var tab = $('ul.tab li',tabArea);
var tabSet = function(target){
var targetTabId = $('a',target).attr('hash');
tab.removeClass('active');
tabPanel.hide();
$(target).addClass('active');
$(targetTabId).show();
}
tab.click(function(){
tabSet(this);
return false;
}).each(function(){
if($(this).hasClass('active')){
tabSet(this);
}
});
if(tabPanel.filter(':visible').length!=1){
tab.eq(0).addClass('active');
tabPanel.not(':first').hide();
}
});
このスクリプトをメソッドにすればいいだけの話なのです。
function関数にする
メソッドにする前に、
function tabPanel(target){ // 引数で対象要素を渡す
var tabArea = $(target); // 引数から要素を受け取りjQueryオブジェクトとして保存しておく
var tabPanel = $('div.tabPanel',tabArea);
var tab = $('ul.tab li',tabArea);
var tabSet = function(target){
var targetTabId = $('a',target).attr('hash');
tab.removeClass('active');
tabPanel.hide();
$(target).addClass('active');
$(targetTabId).show();
}
tab.click(function(){
tabSet(this);
return false;
}).each(function(){
if($(this).hasClass('active')){
tabSet(this);
}
});
if(tabPanel.filter(':visible').length!=1){
tab.eq(0).addClass('active');
tabPanel.not(':first').hide();
}
}
上から2行を見てください。対象にする要素を引数で渡すことができます。ただし、
jQuery(function($){
tabPanel('div.tabArea');
});
jQueryオブジェクトのメソッドにする
では準備ができたところで、
(function($){
// メソッドの登録
$.fn.tabPanel = function(){
// ここに処理を書く
// この中では渡したjQueryオブジェクトをthisとして扱うことができる
}
})(jQuery);
// メソッドの実行
jQuery(function($){
$('div.tabArea').tabPanel();
});
上記の例では、
(function($){
$.fn.tabPanel = function(){
var tabArea = $(this); // this == $(object)
var tabPanel = $('div.tabPanel',tabArea);
var tab = $('ul.tab li',tabArea);
var tabSet = function(target){
var targetTabId = $('a',target).attr('hash');
tab.removeClass('active');
tabPanel.hide();
$(target).addClass('active');
$(targetTabId).show();
}
tab.click(function(){
tabSet(this);
return false;
}).each(function(){
if($(this).hasClass('active')){
tabSet(this);
}
});
if(tabPanel.filter(':visible').length!=1){
tab.eq(0).addClass('active');
tabPanel.not(':first').hide();
}
}
})(jQuery);
// 実行する
jQuery(function($){
$('div.tabArea').tabPanel();
});
機能の拡張
1)複数のタブパネルに対応する
メソッド化までが終わりました。これでプラグインは完成・・・ではなく、
複数の要素を含んだjQueryオブジェクトに対して、
注意しておくべき点は、
(function($){
$.fn.tabPanel = function(){
var tabArea = $(this); // this == $(object)
// eachメソッドを使って複数のjQueryオブジェクトに対しても処理を実行する
tabArea.each(function(){
var tabPanel = $('div.tabPanel',this); // eachメソッドの中ではthisが変わる
var tab = $('ul.tab li',this);
var tabSet = function(target){
var targetTabId = $('a',target).attr('hash');
tab.removeClass('active');
tabPanel.hide();
$(target).addClass('active');
$(targetTabId).show();
}
tab.click(function(){
tabSet(this);
return false;
}).each(function(){
if($(this).hasClass('active')){
tabSet(this);
}
});
if(tabPanel.filter(':visible').length!=1){
tab.eq(0).addClass('active');
tabPanel.not(':first').hide();
}
});
}
})(jQuery);
jQuery(function($){
$('div.tabArea').tabPanel();
});
当然、
2)タブ切り替え時にエフェクトを追加するオプションを実装する
現在のタブパネルメソッドは、
引数に入る値が、
(function($){
$.fn.tabPanel = function(option1,option2){
var show = option1 ? option1 : 'show';
var duration = option2 ? option2 : null;
alert(show);
alert(duration);
}
})(jQuery);
jQuery(function($){
$('div.tabArea').tabPanel('fadeIn',400);
});
もし、
(function($){
$.fn.tabPanel = function(options){
var conf = $.extend({
show: 'show',
duration: null // nullは空という意味
},options || {}); // optionsに値があれば上書きする
alert(conf.show);
alert(conf.duration);
}
})(jQuery);
// オプションの渡し方
jQuery(function($){
$('div.tabArea').tabPanel({
show: 'fadeIn',
duration: 400
});
});
showとhideに、
後は値によって、
メソッドの呼び出し方
メソッドの呼び出し方は、
$(object).fadeIn(400);
しかし、
$(object)['fadeIn'](400);
どちらでも同じ意味です。後者では、
// conf.show: 'show'
// conf.duration: null
$(object)['show']();
// これは $(object).show()と同じ
// conf.show: 'fadeIn'
// conf.duration: 400
$(object)['fadeIn'](400);
// これは $(object).fadeIn(400)と同じ
あとは、
(function($){
$.fn.tabPanel = function(options){
var tabArea = $(this);
var conf = $.extend({
show: 'show',
duration: null
},options || {});
tabArea.each(function(){
var tabPanel = $('div.tabPanel',this);
var tab = $('ul.tab li',this);
var tabSet = function(target){
var targetTabId = $('a',target).attr('hash');
tab.removeClass('active');
tabPanel.hide();
$(target).addClass('active');
$(targetTabId)[conf.show](conf.duration); // 書き換えた
}
tab.click(function(){
tabSet(this);
return false;
}).each(function(){
if($(this).hasClass('active')){
tabSet(this);
}
});
if(tabPanel.filter(':visible').length!=1){
tab.eq(0).addClass('active');
tabPanel.not(':first').hide();
}
});
}
})(jQuery);
jQuery(function($){
$('div#tabArea1').tabPanel();
$('div#tabArea2').tabPanel({
show: 'fadeIn',
duration: 300
});
$('div#tabArea3').tabPanel({
show: 'slideDown',
duration: 200
});
});
以上でタブパネルプラグインが完成しました。オプションでfadeInを渡さずに、